Inverted Kinematics and Pathing with Processing
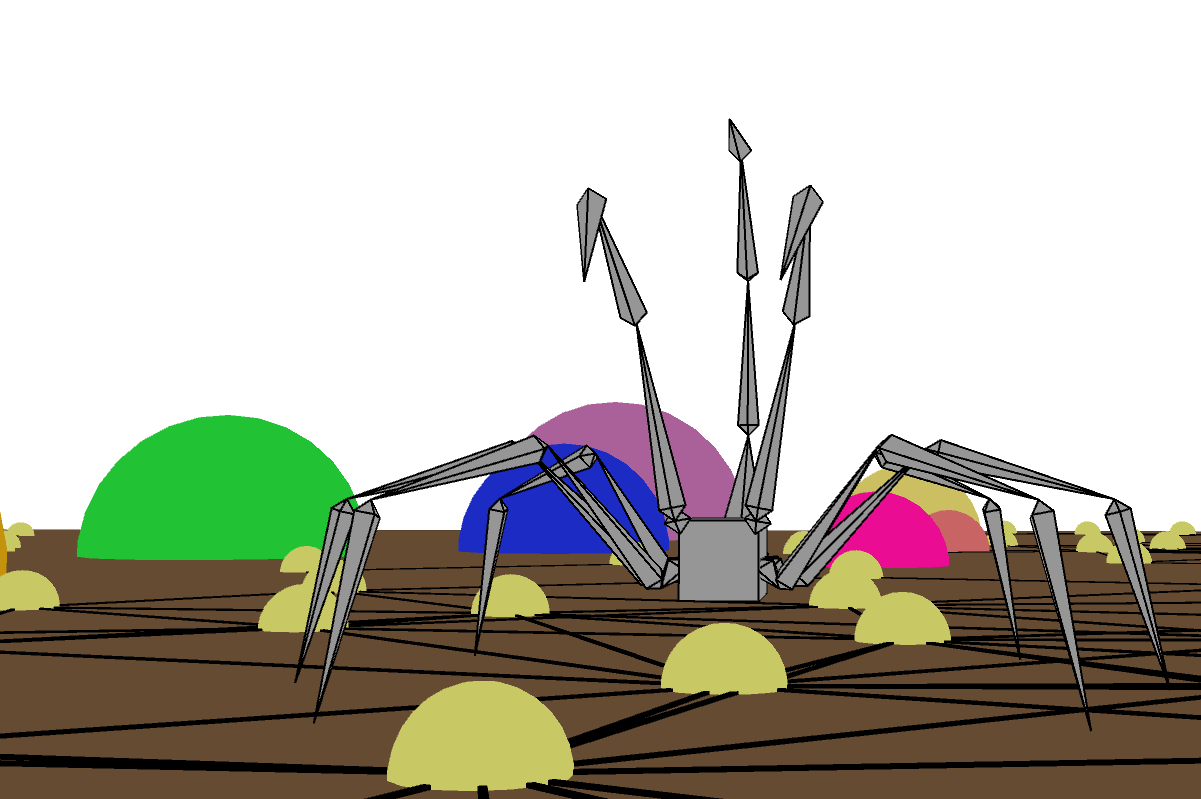
This project was a combination of two smaller simulations as the third major assignment in CSCI 5611 - Animation and Planning in Games.
This assignment was a bit rough for me, mostly because I attempted to combine both aspects of the project into one sketch. Because I wanted to do the whole thing with 3D rendering, this meant that the kinematics example I was looking at wouldn't translate well given the method I used for creating my agent model. I ended up spending a significant amount of time trying to get it to work, only to have to reverse course and simply submit a separate file for the IK simulation.
That being said, I did end up with a neat looking model that articulates, just not well, and not with IK.
Sketches
Inverse Kinematics
For this sketch, we were tasked with creating a multi-node arm that could easily reach through a 2D plane. This part of the project was scaffolded off of the IK_1Frozen.pde
example file provided in class.
-
Single-arm IK - This is the basis for this sketch. Allow a single arm with at least four joints to move smoothly in the simulation. The example file started with three joints (including the root) and three segments. Once all the variables were placed into arrays, it was easy enough to extend the number of joints.
-
Joint Limits - The second requirement of this simulation. Joints should only be allowed to rotate a specific amount in either dimension. Since the geometry of the arms is fairly uniform, and the baseline for each is along the axis of reflection for each piece, each segment of the arm is allowed to deflect in either direction up to a pre-defined amount. These values were hard-coded in an array for simplicity, since this was after the formal submission date.
-
User Interaction - I was also lucky enough to start from a file that draws a circle around the mouse pointer. The arm then reaches for the circle without the user needing to do anything more than hover their mouse.
"Crowd" Simulation
This was the basis for what I wanted to do for the whole project. The idea was to take the professor's PRM_Exercise_SingleFile.pde
and convert it into 3D, adding on top of it an agent that could reach towards an actual goal. When creating the skeleton of the model, I went with the method that each bone's rotation and placement were based on their parent's. This is fine when the motion is controlled from the root out to the extremities, but IK does this backwards, needing the ability to set the positions of the parents end effectors. Since the actual end effectors in this system are derived from their rotational geometries, the math involved to get IK to work, as the professor mentioned, "Several weeks of development."
So, here we are, a pretty model that does not include IK, but at least can kinda traverse a randomly generated graph of a continuous space.
-
Single Agent Navigation - There is a single agent, and it navigates it's environment. There is no explicit collision detection here since this can all be handled by the way that the map is generated. No paths are drawn between obstacles that would cause the bounding boxes to overlap. All of the obstacles here are spheres, but their bounding geometries use axis-aligned boxes, in the scenario where more complex models would be used. An example of the close pathing can be seen towards the end of the video.
-
Motion Planning - I stuck with the example PRM model since I clearly didn't need more challenges to get this project up and running. All of the small, light-yellow domes are the nodes of the graph. All the black lines between them are valid links. Links are restricted to be within a specific length and nodes are encouraged to space out slightly. No nodes are generated inside of bounding geometries for the obstacles. No paths are generated for connections that would cause the agent's bounding box to overlap with the other boxes. The agent uses simple breadth-first search, same as the original example.
-
3D Rendering & Camera - This part was thanks again to the use of Liam Tyler's camera class as well as the additions made by the professor to select items in 3D space. The camera is placed at an angle to show of the 3D nature, but the previous controls still work. With the professor's additions, you can click about the floor to select a point for which the agent will do their best to navigate to.
Code Pack
For grading purposes, the code is available through my university Google Drive folder.
Resources
- In a moment of frustration, all of the vectors went away from the manual implementations we were using in prior projects into Processing's own
PVector
class. They are roughly the same, but I was getting more consistent results. - Along with normal Java classes and functions, I utilized some
Math
library calls. - The
Camera.pde
class for the "crowd" simulation was written by Liam Tyler and adapted by professor Guy to include a 3D pointer. - Professor Guy's example files for
IK_1Frozen.pde
andPRM_Exercise_SingleFile.pde
were the basis of these sketches. - This article was also of great help for getting the ray and AABB collision working correctly.
Closing Remarks
There were many small things that consumed time and made the possibility of doing what I had desired no longer feasible, which is a generally frustrating feeling. I felt like I'd spend several hours debugging a thing, only to start with a fresh slate and copy the same code over to find it suddenly working without issue. I don't claim that this was any kind of black magic, but I clearly ended up too deep in forest to see the trees frequently on this assignment, and only the most frustrating example was trying to implement IK through rotational and relative geometries.
I think had I started from the IK example first and worked my way backwards from what I ended up doing, I may have been able to better plan out a structure that included better IK-safe algorithms that would work okay in the environment we were given. I would have liked also to take a stab at more collision detection methods with either AABB or more complex detection models, but that may have to be something I explore in my free time.
- Java
- Processing
- Motion Planning